Version Overview
ASN Filter Designer’s DSP ANSI C SDK framework provides embedded developers with a comprehensive C SDK for their IoT microcontroller projects. This allows developers to directly deploy an IoT filtering application from within the tool to any STM32, Arduino, ESP32, PIC32, Beagle Bone and other microcontrollers for direct use.
The following table summarises the current versions of the SDK depending on the version of tool being used.
SDK | ASNFD | Description | Doc |
v2.x | v5.4.0 and higher | The DSP ANSI C v2.x SDK provides significant improvements over v1.x, with strict data typing, improved memory management, and support for single-sample and multi-sample modes — specifically designed to help Arm developers implement reliable real-time embedded systems very easily. |
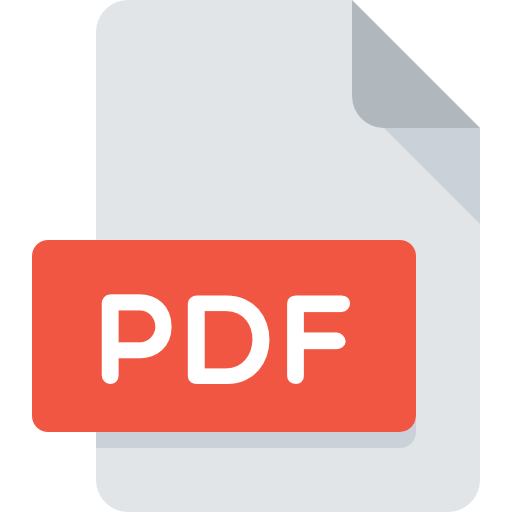
|
v1.x | v5.0 - v5.3.5 | The DSP ANSI C v1.x SDK is a legacy SDK for use with ASN Filter Designer up to version 5.3.5. It is not maintained and is not recommended for new designs. |
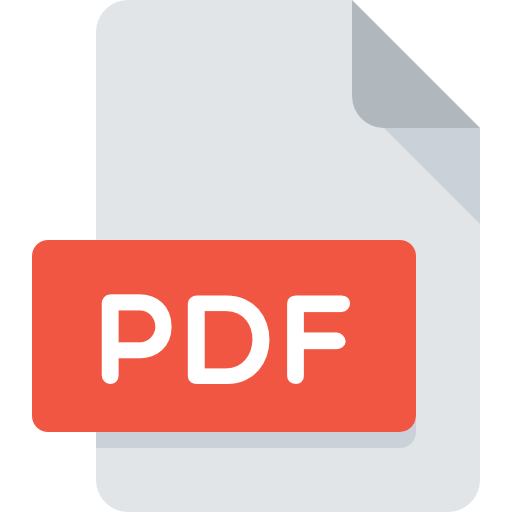
|